So, What Exactly Is NodeJS?
Node.js is a powerful JavaScript runtime that has become a staple of web development since it came out in 2009.
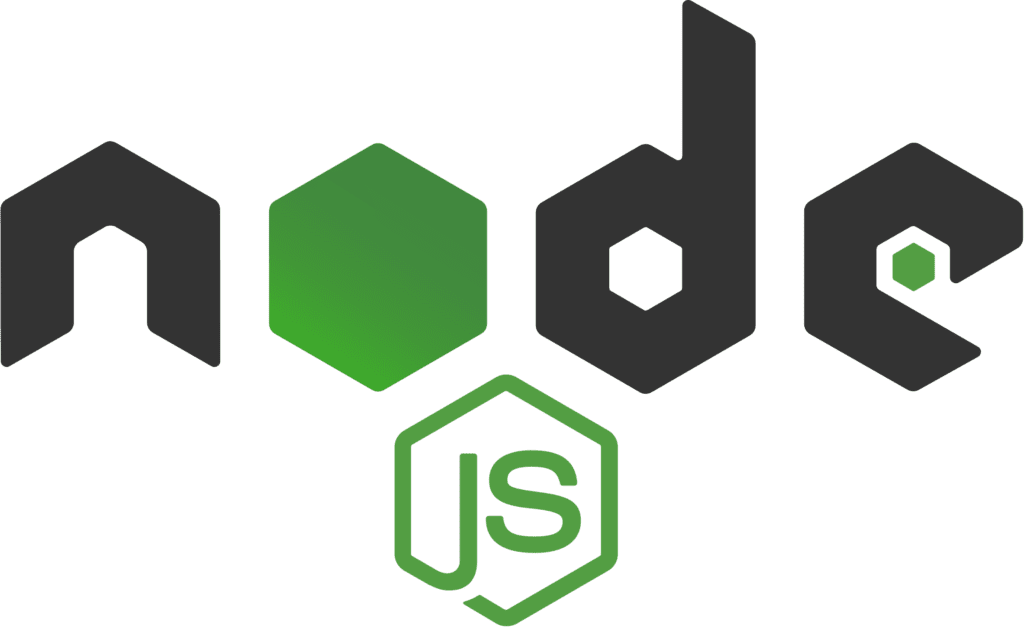
This guide aims to provide developers and founders with the necessary information to determine if Node.js is the right choice for their project in 2024.
An Overview of Node.js
Here’s the official description from Node.js.
Node.js® is a platform built on Chrome’s JavaScript runtime for easily building fast, scalable network applications. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
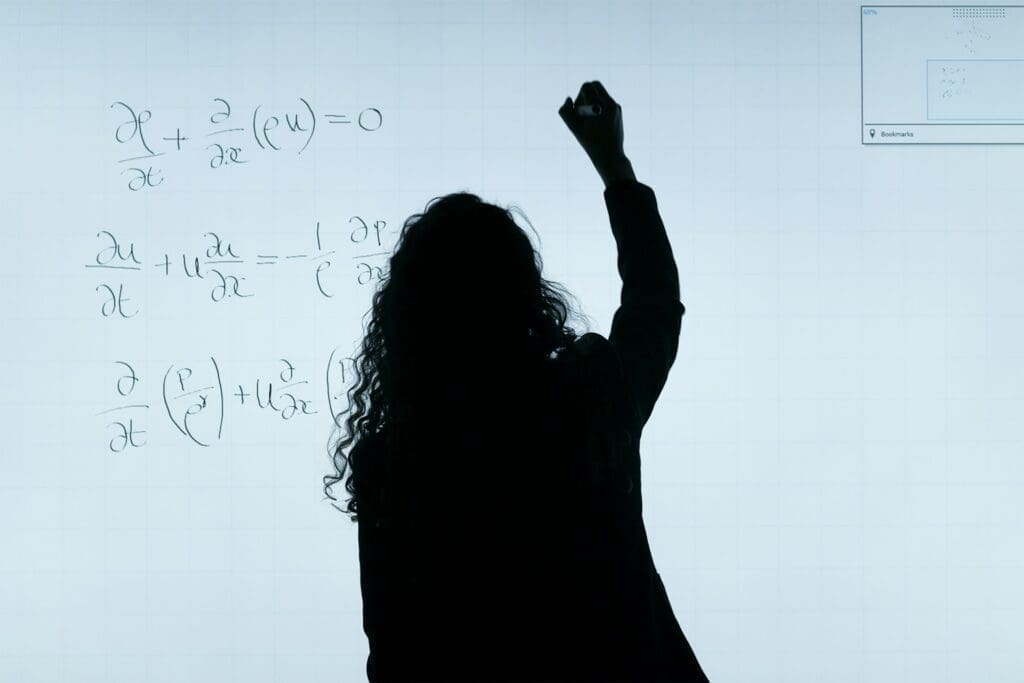
Give whoever wrote that a smack and make them use normal English and you’ll get this:
Node.js is a completely free and customizable tool that helps run web apps on different devices, not just in web browsers.
In a nutshell, the primary advantage of NodeJS is that it allowed developers who knew JavaScript – a frontend development language – to write backend services.
If nothing else, remember that!
If you’d like to know more, let’s dive into why Node.js is a valuable tool for the web development community.
Key Node.js Terminology
To any non technical founders reading this guide, here are some definitions of key terms regarding Node.js that will make it easier to understand the later sections.
If you’re a developer, feel free to skip this!
Term | Definition |
JavaScript (JS) | A widely-used programming language for client and server-side development. |
Server-Side JavaScript | Running JavaScript code on the server to handle server-related tasks, such as processing requests and managing databases. |
Runtime | The environment where a programming language’s code is executed. |
Asynchronous | Different tasks can be performed independently. |
Non-Blocking I/O | Tasks can begin without waiting for one to complete before starting another. |
Event-Driven | Where actions or occurrences (events) trigger responses. |
Callback Function | A way to make sure the program knows what to do when a specific task is done, especially when a task takes time to complete. |
Package Manager | A tool for managing and installing third-party libraries (packages) in Node.js projects. |
Express.js | A popular web application framework for Node.js. |
Middleware | Software that sits between an application and the server, handling tasks like authentication and user login. |
RESTful API | A web service commonly used in Node.js for building APIs. |
WebSocket | Enables real-time, bidirectional communication between clients and servers, often used for building interactive applications. |
V8 Engine | Google’s open-source JavaScript engine that powers Node.js, responsible for executing JavaScript code. |
libuv | A cross-platform library used by Node.js to handle asynchronous I/O operations, such as file system access. |
Top 3 Advantages of Using Node.js
While not perfect, the fact that Node.js has been used for so long tells you it must have plenty of advantages, and we’ve listed some major ones in this section.
Hope you skimmed through our vocabulary list – many of those terms appear below!
1. Reduced Complexity for Web Developers
Different programming languages have their own advantages, and JavaScript’s strength has always been frontend web development.
For a long time, JavaScript was mainly used in web browsers, and you couldn’t really use it to create things like a web server or anything beyond what could run in a browser.
Instead, you had to use other languages like Java, C#, Go, or C for those tasks.
Node.js changed this by using Google’s V8 engine (written in C++) to expand where you could use JavaScript.
Now, it can be used in any situation just like other languages.
2. Reduced Dependency on Other Tools
Because Node.js can run HTTP applications written in JavaScript, it can also emulate other programs such as Apache’s httpd which has led to the rise of isomorphic web applications written in JavaScript.
An isomorphic web application uses JavaScript both in the browser and on the server, and using the same language allows for code sharing which reduces testing and maintenance time.
3. Asynchronous Nature
By asynchronous, we mean that Node.js can handle multiple tasks at the same time without making one wait for the other to finish.
In many languages, the program moves on to the next only when the current one is done.
This makes coding in JavaScript different, but some find it advantageous, especially due to its ability to handle many tasks simultaneously without delays.
Other languages may not have this feature, leading to what developers sometimes call “callback hell.”
When to Use Node.js
Because of its single-threaded approach (handle multiple tasks without waiting for one to finish before starting another) Node.js excels in use cases where it can efficiently manage non-blocking and event-driven servers, which means environments with:
- real-time data
- asynchronous tasks
- small, independent, and scalable services, and
- serverless computing
With that in mind, here are some common use cases for Node.js:
Traditional Websites
Its non-blocking nature allows it to serve a large number of users simultaneously, and Node.js is particularly effective for websites with real-time features like instant messaging or live updates, thanks to its event-driven model.
Above all else, developers can use JavaScript on both the frontend and backend, promoting code reuse and shortening development time – hooray!
Streaming Services:
Node.js is suitable for building streaming applications, such as video and audio streaming services, due to its ability to handle real-time data efficiently.
Back-End API Services
Node.js can efficiently handle a large number of concurrent connections without waiting for one task to finish before moving on to the next.
This makes it ideal for processing multiple requests simultaneously, exactly what your average API service is expected to handle.
Collaboration Tools
Because the best collaborative editing tools and project management platforms offer users real-time collaboration, they benefit tremendously from Node.js’s ability to handle concurrent connections and events without clogging up.
Microservices
Node.js is well-suited for building microservices, which are small, independent, and scalable services that work together to form a larger application.
Cross-Platform Desktop Applications
Tools like Electron leverage Node.js for building cross-platform desktop applications. Electron enables the development of applications using web technologies (HTML, CSS, JavaScript) that run on Windows, macOS, and Linux.
Alternative Web Development Tools to Node.js
While Node.js is a strong contender, other back-end technologies like Python, Ruby on Rails, and Java have their unique strengths. Here’s a table comparing Node.js with common alternatives:
Framework | Language | Strengths | Use Cases |
---|---|---|---|
Ruby on Rails | Ruby |
|
|
Django | Python |
|
|
Express.js | JavaScript |
|
|
Spring Boot | Java |
|
|
Laravel | PHP |
|
|
Flask | Python |
|
|
ASP.NET Core | C# |
|
|
The choice of framework depends largely on your development team’s expertise.
We will say, however, that Node.js excels at handling asynchronous I/O operations and large volumes of requests which make it superior in certain use cases.
npm: The Heart of the Node.js Ecosystem
npm serves as a centralized hub for countless packages and modules.
Ok, you can technically count them, but it’s a really big number and who has time for that?
Anyways, this streamlines the process of integrating third-party solutions into Node.js projects, making it super easy for developers to manage and share code.
Streamlining Development
npm allows developers to effortlessly install, update, and manage dependencies, making the development process more efficient.
With a vast repository of open-source packages, developers can leverage existing solutions, avoiding the need to reinvent the wheel for common functionalities.
Version Control and Collaboration
npm facilitates version control, ensuring that projects remain consistent and compatible across different environments.
This feature is pivotal for collaborative development, enabling teams to work seamlessly on a shared codebase without worrying about compatibility issues.
Leveraging Packages and Modules
Modular Development
One of the key advantages of Node.js is its emphasis on modular development.
Developers can break down their applications into smaller, manageable modules, each serving a specific purpose. npm simplifies the integration of these modules.
This promotes code reusability and maintainability.
Dependency Management
Developers can declare project dependencies in a package.json file, specifying the required packages and their versions.
npm then automatically installs and manages these dependencies, ensuring that the project can be easily replicated on different machines.
Popular Libraries and Frameworks
This section is more for the developers reading, but non tech founders are welcome to enjoy the 1s and 0s too!
Express.js
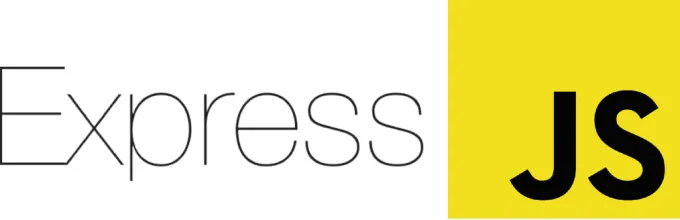
Description: A minimalistic web application framework for Node.js, Express.js simplifies the creation of robust and scalable web applications.
Application: Building RESTful APIs, web servers, and dynamic web applications.
Socket.io
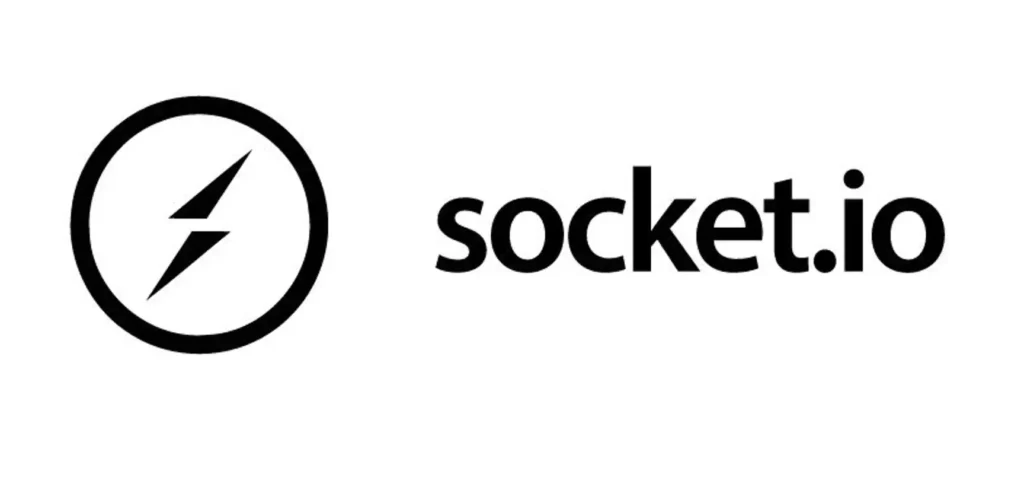
Description: A library enabling real-time, bidirectional communication between clients and servers using WebSockets.
Application: Real-time chat applications, online gaming, live updates in collaborative environments.
Mongoose
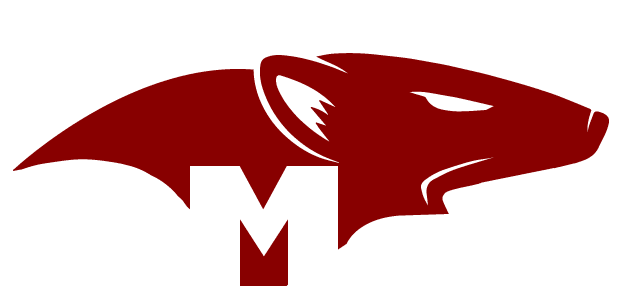
Description: An elegant MongoDB object modeling tool for Node.js, simplifying interactions with MongoDB databases.
Application: Developing database-driven applications, handling database operations seamlessly.
Timeless Node.js Development Resources
- Official Documentation:
- Community Forums:
- Interactive Tutorials:
- YouTube Channels:
- Books:
- “Node.js Design Patterns” by Mario Casciaro
- “Node.js Web Development” by David Herron
Node.js 2024 Trends
Staying ahead in web development means keeping a close eye on trends and advancements in Node.js.
Before we can predict the future, let’s look at some key present-day statistics.
- Over 6.3 million websites use Node.js in the U.S., making it the country’s most widely used web development tool.
- Netflix and PayPal enjoyed reduced loading time by 50% to 60% with Node.js.
- Utilizing Node.js development, on average, reduces development costs by 58%.
- 42.73% of pro developers prefer popular Node.js frameworks, libraries, tools, and IDEs.
- Over a quarter million companies use Node.js as a programming language.
Based on present-day statistics, here are three likely trends for Node.js.
Increased Adoption Across Industries
The widespread usage of Node.js by websites in the U.S. suggests a continued upward trend in its adoption across various industries.
As more companies experience the benefits, we expect an increased embrace of Node.js in diverse sectors.
Increased Adoption by Developers and Continued Ecosystem Growth
The fact that 42.73% of professional developers prefer popular Node.js frameworks, libraries, tools, and IDEs shows how strong the Node.js developer community is.
This trend is likely to continue, leading to the growth of the Node.js ecosystem.
As more developers embrace and endorse Node.js, we anticipate new tools, libraries, and frameworks that make development faster and more efficient.
Optimization for Performance and Cost Reduction:
The success stories of Netflix and PayPal, showcasing a 50% to 60% reduction in loading time, highlight the importance of performance optimization.
The emphasis on reducing development costs by 58% further suggests a trend toward prioritizing frameworks that enhance performance and cost-efficiency.
This leverages Node.js for efficient and cost-effective web development.
Choosing the Right Node.js Development Team
Choosing the right Node.js development team is crucial.
Look for a team with extensive experience, technical expertise, and a robust portfolio.
It’s equally important to find a team that aligns with your company culture and shares your vision for the project.
Additionally, the popularity of Node.js allows you to consider offshore developers, which offers tremendous cost-saving opportunities with minimal added risk when done right.
Conclusion
With its dynamic features and robust ecosystem, Node.js can help deliver high-quality web applications.
However, using Node.js in your web development project should be an informed decision.
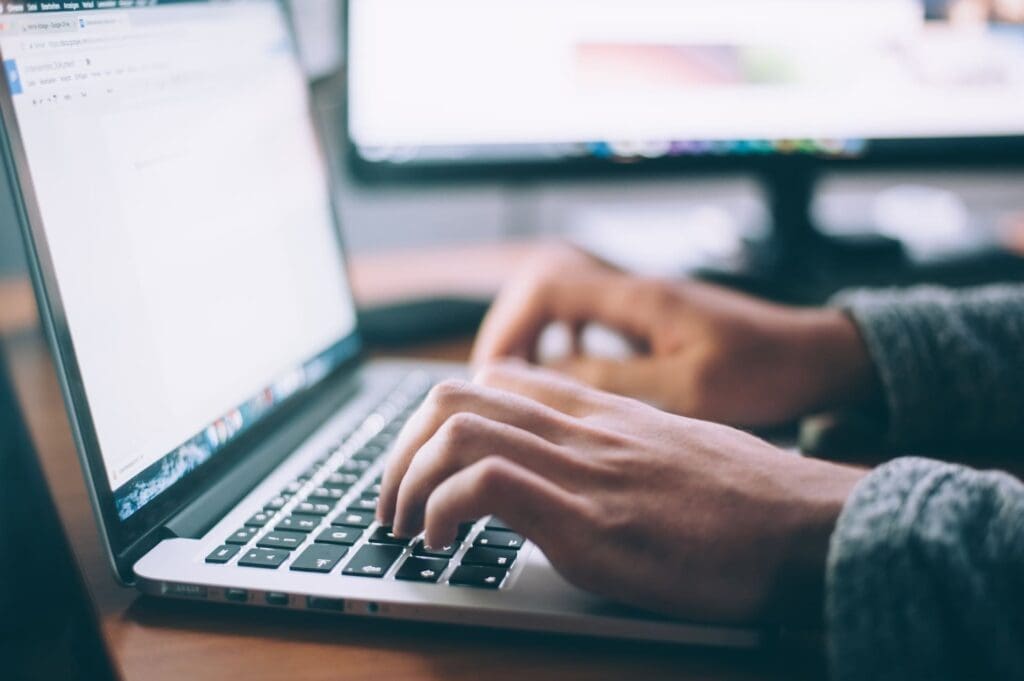
As with any tool, we recommend due diligence and gaining a basic understanding of several popular web development frameworks for a truly informed choice – in which case, you’ll want to engage app developers with the necessary experience.